6 Smarter Ways to Write Conditionals In React You Should Know About
Created By /
heymmusttech.devReading time /
4 Min
Category /
front-end
Date /
16 Jan 2025
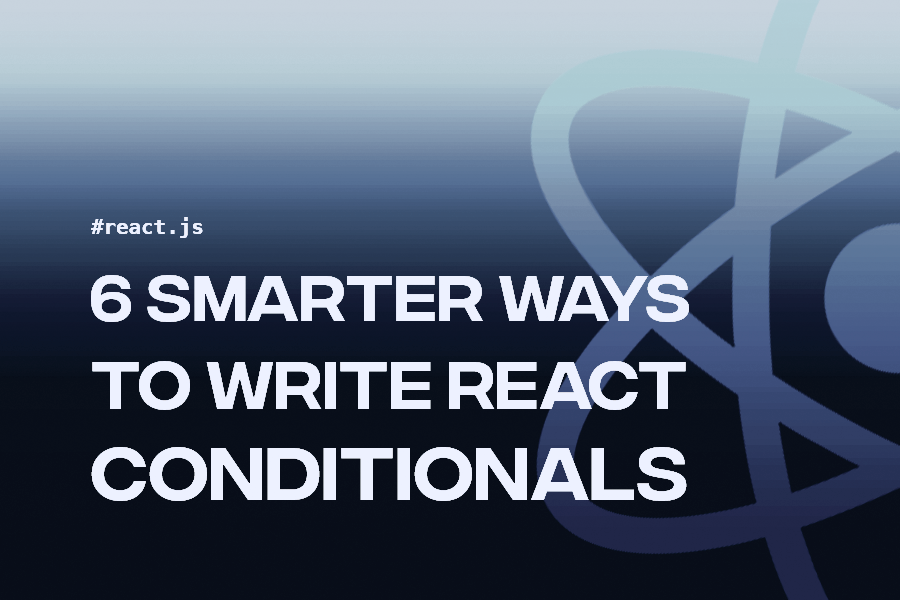
That's a terrible problem
In React, we often write conditional statements to display different views, Like this simple example.
const App = () => {
return (
<>
{
loading ? <Loading /> : <List>
}
</>
)
}
But when the code size of the project is large enough and there are a lot of JSX conditionals, things can quickly get out of hand. The code becomes extremely messy and poorly readable.
Like this code below, I really don't have the courage and confidence to know its details…
import React, { useState } from "react"
export default function ConditionDemo() {
const [ loading ] = useState(false)
const [ isLogin ] = useState(true)
const [ hasAuth ] = useState(false)
return (
<>
{
loading ?
<div className="loading">
loading
</div> :
<div className="content">
{
isLogin ?
(
hasAuth ?
<div className="has-permission">
has-permission
</div> :
<div className="no-permission">You have no permission to operate</div>
) :
<div className="go-login">Please login first</div>
}
</div>
}
</>
)
}
So in React, what are the ways we can write more readable and maintainable code?
Use the ternary operator
The ternary operator is more suitable for scenarios that require a small number of conditional judgments.
If there are many conditional branches, the disaster of the above example will occur.
For example, to display a Mobile
component on a mobile device and another component on other screens, you can use a ternary expression to achieve:
export default function AppTernaryExpressions() {
const isMobilePhone = truereturn (
<>
{ isMobilePhone ? <MobileComp /> : <PcComp /> }
</>
)
}
Use "&&" to simplify the ternary operator
Sometimes we can use "&&" to simplify ternary expressions, such as the following code.
show ? <ShowComp /> : null
&&
!!show && <ShowComp />
Why use two exclamation marks to convert the show to a boolean value? You are welcome to check out my other article with very detailed instructions.
Use "if" statement
If there is data, the List
component is displayed. If there is no data, nothing is displayed. Using the if
statement is also a good choice.
export default function AppIf() {
const { list } = useList()
if (!list) {
return null
}
return (
<List list={list} />
)
}
Of course, sending a request does not always succeed and may fail. We can add some if
branches to control different logic.
export default function AppIf() {
const { isLoading, isError, list } = useList()
if (isLoading) {
return <div>Loading...</div>
}
if (isError) {
return <div>Error...</div>
}
return <List list={list} />
}
Use "switch"
Too many if
statements can lead to cluttered components. Multiple conditions can be extracted into separate components containing switch
statements.
Let's take a look at a simple menu toggle component:
const MenuItem({ menu }) => {
switch (menu) {
case 1:
return <Users />
case 2:
return <Chats />
case 3:
return <Rooms />
default:
return null
}
}
export default function Menu() {
const [menu, setMenu] = React.useState(1)
const toggleMenu = () => {
setMenu((m) => {
if (m === 3) return 1return m + 1
})
}
return (
<>
<MenuItem menu={ menu } />
<button onClick={ toggleMenu }>toggle menu</button>
</>
)
}
You can see that using 'switch' can easily express the corresponding relationship between 'menu' and components.
Use enumerations
If we need to display the three components Foo
, Bar
, and Default
according to the user's different states, enumeration will be more elegant than the if
statement.
const Foo = () => {
return <div>foo</div>
}
const Bar = () => {
return <div>bar</div>
}
const Default = () => {
return <div>default</div>
}
const statusMap = {
foo: <Foo />,
bar: <Bar />,
default: <Default />
}
const App = () => {
const [status] = useState('default')
return (
statusMap[status]
)
}
Use JSX Control Statements
The JSX control statements library extends the functionality of JSX so that you can directly use JSX to implement conditional rendering.
Let's take an example.
export default function App(props) {
const [ hasLogin ] = useState(false)
return (
<Choose>
<When condition={ hasLogin }>
<button>Logout</button>
</When>
<When condition={ !hasLogin }>
<button>Login</button>
</When>
</Choose>
)
}
Finally
Thanks for reading. I am looking forward to your following and reading more high-quality articles.